API
API documentation for our developer-first translation management platform
API vs. CLI
This documentation covers the RESTful i18nexus API. While it's fully featured, many teams prefer using our CLI (Command Line Interface) for easier access, especially when downloading translations during their app's build process.
CLI documentation is available here: i18nexus CLI
Authorization
The Project Resources API is a simple RESTful API for accessing your i18nexus project data. This API is located at the following base URL:
https://api.i18nexus.com/project_resources/
By default, all read-only endpoints can be accessed by including your project API key in the request. For example:
https://api.i18nexus.com/project_resources/translations.json?api_key=:your_api_key
For API endpoints that write project data, a Personal Access Token is required in the header of the request:
Authorization: Bearer YOUR_TOKEN
Tokens are created per user with a scope limited to that of the user's role on the project. They are created in a user's account settings in the i18nexus Dashboard:
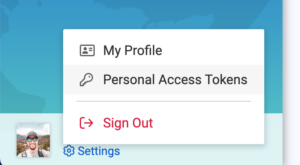
Get Project Resources
GET Translations: By language and namespace
Returns a JSON object of a project’s translations for a specified language and namespace.
Optionally include param confirmed=true
to retrieve only your confirmed translations
GET /project_resources/translations/:language_code/:namespace.json
{
"welcome": "Welcome to my app!",
"goodbye": "Thanks for using my app!"
}
GET Translations: By language
Returns a JSON object of a project’s translations for all namespaces for a single language.
Optionally include param confirmed=true
to retrieve only your confirmed translations
GET /project_resources/translations/:language_code.json
{
"my-first-namespace": {
"welcome": "Welcome to my app!",
"goodbye": "Thanks for using my app!"
},
"my-second-namespace": {
"greeting": "How are you?"
}
}
GET Translations: All
Returns a nested JSON object of all project translations for all languages and namespaces.
GET /project_resources/translations.json
{
"en": {
"my-first-namespace": {
"welcome": "Welcome to my app!",
"goodbye": "Thanks for using my app!"
},
"my-second-namespace": {
"greeting": "How are you?"
}
},
"es-MX": {
"my-first-namespace": {
"welcome": "¡Bienvenido a mi aplicación!",
"goodbye": "¡Gracias por usar mi aplicación!"
},
"my-second-namespace": {
"greeting": "¿Cómo estás?"
}
}
}
GET Versions
Returns a collection of a project’s exported versions ordered from newest to oldest.
By default the latest 10 versions will be returned, but this can be adjusted using the optional limit
query parameter.
GET /project_resources/versions.json?limit=10
{
"collection": [
{
"created_at": 1631402817503,
"description": "My version description",
"download_url": "https://cdn.i18nexus.com/versions/1/translations.zip",
"id": "c3ee93c5-66c8-487d-8cbb-0e6109550f35",
"key_separation": true,
"languages": ["en","es-MX"],
"namespaces": ["my-first-namespace", "my-second-namespace"],
"version_number": 1
}
]
}
GET Namespaces
Returns a collection of a project’s namespaces.
GET /project_resources/namespaces.json
{
"collection": [
{
"id": "c3ee93c5-66c8-487d-8cbb-0e6109550f35",
"title": "common",
"created_at": 1631402817503,
},
{
"id": "77915b03-496d-4c48-83c0-cece670ff5b3",
"title": "homepage",
"created_at": 1633402920418
}
]
}
GET Languages
Returns a collection of a project’s languages.
GET /project_resources/languages.json
{
"collection": [
{
"name": "English",
"full_code": "en",
"language_code": "en",
"country_code": null,
"google_translate_code": "en",
"base_language": true
},
{
"name": "Spanish",
"full_code": "es",
"language_code": "es",
"country_code": null,
"google_translate_code": "es",
"base_language": false
}
]
}
Write Project Resources (Advanced)
Almost all i18nexus users prefer using the following APIs through the i18nexus-cli, where each request is wrapped in a simple command. Direct access to the following APIs is meant for teams who want to integrate i18nexus into their own management systems and workflows.
Reminder: Write APIs are only authorized using Personal Access Tokens.
Create String
Creates a new string in your project’s base language, and creates machine translations for each of your project’s languages.
Parameters:
Property | Type | Note | Required? |
---|---|---|---|
key | string | The key of the string to create | |
value | string | The value of the string to create | |
details | string | The details of the string to create | |
ai_instructions | string | Instructions or context for AI machine translator | |
namespace | string | The namespace in which to create the string |
Example Request Body:
POST /project_resources/base_strings.json
{
"key": "welcome_msg",
"value": "Welcome to my app!",
"details": "Appears in the home page header.",
"ai_instructions": "Make it informal and keep it less than 5 words"
"namespace": "homepage"
}
Update String
Updates a string’s key, value, details, and/or namespace.
Parameters:
Property | Type | Note | Required? |
---|---|---|---|
id | object | An object containing the key and namespace of the string to update | |
key | string | The new key | |
value | string | The new value | |
details | string | The new details | |
ai_instructions | string | Instructions or context for AI machine translator | |
namespace | string | The namespace in which to move the string | |
reset_confirmed | boolean | Required if updating the value of the string and the string contains confirmed translations. Determines whether or not to reset the values of confirmed translations with machine translations of the new value. |
Example Request Body:
In this example we are changing the key and the value of the string with key notifications
in namespace common
.
PATCH /project_resources/base_strings.json
{
"id": {
"key": "notifications",
"namespace": "common"
},
"key": "notifications.alert",
"value": "You have a new notification!",
"reset_confirmed": true
}
Delete String
Deletes a string and its associated translations in your project.
Parameters:
Property | Type | Note | Required? |
---|---|---|---|
id | object | An object containing the key and namespace of the string to delete |
Example Request Body:
In this example we are deleting the string with key notificiations.alert
from namespace common
.
DELETE /project_resources/base_strings.json
{
"id": {
"key": "notifications.alert",
"namespace": "common"
}
}
Import Strings from JSON
Imports a block of JSON key-value pairs into your project. This is the equivalent of using the Import tool in the Strings Management page.
Parameters:
Property | Type | Note | Required? |
---|---|---|---|
languages | object | JSON block of strings with language codes as the keys (see example request body below) | |
namespace | string | The namespace to import into | |
confirm | boolean | If importing any translation strings (strings for a language that is not the project base language), mark them "confirmed" in i18nexus (default: false) | |
overwrite | boolean | If any keys already exist in the target namespace, overwrite the values with the imported values (default: false) |
Example Request Body:
In this example we are importing strings into our project’s common
namespace for English (en) and Spanish (es).
You do not have to include all of your projects’ languages in your request, only the ones you wish to update.
POST /project_resources/import.json
{
"namespace": "common",
"confirm": true,
"overwrite": true
"languages": {
"en": {
"welcome_msg": "Welcome to my app!",
"sign_in": "Sign In",
"sign_out": "Sign out",
"notifications": {
"alert": "You have a new notification!"
}
},
"es": {
"welcome_msg": "¡Bienvenido a mi aplicación!",
"sign_in": "Iniciar sesión",
"sign_out": "Desconectar",
"notifications": {
"alert": "¡Tienes una nueva notificación!"
}
}
}
}
Limits
To prevent abuse, the i18nexus API has rate limits in place. The following limits are primarily only of concern to those teams who choose to fetch their translations at runtime in their applications. If you have a high traffic application, we recommend downloading and bundling your translations at build time in order to avoid hitting the following limits.
Request Limits (Gauged by API key)
API Fetch Requests | CDN Fetch Requests | |
---|---|---|
Free | 5K/month | 10K/month |
Basic | 30K/month | 100K/month |
Standard | 200K/month | 1M/month |
Pro | 1M/month | 6M/month |
Enterprise (Negotiable) | 1M/month | 6M/month |
Rate Limits (Gauged by IP address)
API Fetch Requests | API Write Requests | |
---|---|---|
All Plans | 100/second | 10/second |
Level up your localization
It only takes a few minutes to streamline your translations forever.
Get started